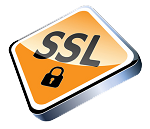
Au cours de la plupart des développements actuels de logiciels exploitant les réseaux, la sécurité de ces échanges est primordiale. Une des solutions les plus employée de part sa facilité d’intégration, sa standardisation et qui a fait ses preuves est une encapsulation via le protocole SSL/TLS.
SSL (Secure Socket Layer) / TLS (Transport Layer Security) est un protocole permettant de relier des systèmes informatiques entre eux d’une manière sécurisée. Garantissant l’intégrité des échanges, la confidentialité et l’authentification des données, ce protocole est un standard en termes de protocole sécurisé. Datant de de 1994, SSL se découpe en 3 versions (1.0 non-utilisée, 2.0 jugée à présent obsolète et insécurisée et 3.0 extrêmement déployée). Après une standardisation du protocole par l’IETF en 2001, SSL à changé de nom pour TLS qui lui aussi se découpe en 3 version (1.0 très déployée, 1.1 et 1.2 qui étendent ses fonctionnalités).
SSL/TLS se découpe en 4 sous-protocoles:
- Handshake : la négociation des paramètres de sécurité en tant que tel.
- Change Cipher Spec : la validation de la négociation préalable, pour vérifier que les deux partis se sont bien accordés quant à la clé maîtresse générée, aux algorithmes à employer etc.
- Alert : protocole informatif de l’état de la liaison.
- Record : protocole d’acheminement des données de la communication. Ce protocole peut encapsuler tout autre protocole et le rend ainsi sécurisé.
SSL/TLS évolue à l’inter-couche transport/application de la pile OSI. Il est utilisé avec d’autres protocoles standardisés tels que HTTP, FTP ou encore SMPT en leur ajoutant un “S” final. Pour les puristes, “HTTPS”, “FTPS” ou “STMPS” ne sont pas des protocoles en tant que tel. Ce sont des encapsulations de protocoles insécurisés (HTTP) dans une couche SSL/TLS ; d’où leur mise en italique dans la figure suivante.
Ce protocole se fonde initialement sur un mode de transport TCP (pouvant être UDP pour le DTLS, WTLS…) et s’implémente dans des développements logiciels par le biais d’API dont certaines des plus connues sont:
- OpenSSL : l’API de référence.
- GnuTLS : alternative à OpenSSL
- yaSSL : version destinée au monde de l’embarqué
- MatrixSSL : cible également le monde de l’embarqué
Au sein de cet article, il vous est présenté une implémentation simple d’un client et d’un serveur exploitant le protocole SSL/TLS pour communiquer via l’API OpenSSL. Ce client/serveur est multi-plateformes Windows/Linux.
Fonctionnalité du serveur :
- Se met en écoute sur un port défini
- Permet d’utiliser les versions SSL2.0, SSL3.0n SSL2.0 & 3.0, TLS1.0
- Permet de charger un certificat contenant une clé publique à partir d’un fichier, idem concernant la clé privée.
- Permet d’utiliser un certificat contenant une clé publique et une clé privée codés en dur dans l’application, au format PEM (encodage base64 du format DER).
- Permet de générer un nouveau certificat et une nouvelle clé publique dynamiquement à chaque lancement du serveur.
- Le serveur vérifie la correspondance entre le certificat et la clé privée avant de se mettre en écoute.
- Le serveur patiente jusqu’à la connexion d’un client. Il affiche les détails du potentiel certificat envoyé par le client (facultatif) et attend la réception d’un message via le protocole “record“.
- Lorsqu’un message arrive, celui-ci est directement retourné au client (ping-pong) puis le serveur attend une nouvelle connexion.
* SSL/TLS server demonstration. This source code is cross-plateforme Windows and Linux.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* Compile under Linux with : g++ main.cpp -Wall -lssl -lcrypto -o main&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* Certificat and private key to protect transaction can be used from :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – External(s) file(s), created with command : openssl req -x509 -nodes -newkey rsa:2048 -keyout server.pem -out server.pem&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – Internal uniq hardcoded certificat and private key, equal into each server instance&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – Randomly generated certificat and private key, best solution to used dynamic keying material at each server lauching.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* Usage :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* # run the server on port 1337 for SSLv2&amp;amp;amp;amp;3 protocol with internals key and certificat&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* $ [./]server[.exe] 1337&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* # run the server on port 1337 for TLSv1 protocol with key and certificat in server.pem file&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* $ [./]server[.exe] 1337 1 server.pem server.pem&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @author x@s&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#define DEFAULT_PORT 443&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#ifdef __unix__ // __unix__ is usually defined by compilers targeting Unix systems&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;unistd.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;sys/socket.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;arpa/inet.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;resolv.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define SOCKLEN_T socklen_t&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define CLOSESOCKET close&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#elif defined _WIN32 // _Win32 is usually defined by compilers targeting 32 or 64 bit Windows systems&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;windows.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;winsock2.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define SOCKLEN_T int&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define CLOSESOCKET closesocket&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#include &amp;amp;amp;lt;stdio.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;errno.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;unistd.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;malloc.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;string.h&amp;amp;amp;gt;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#include &amp;amp;amp;lt;openssl/crypto.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/x509v3.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/pem.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/ssl.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/err.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/bio.h&amp;amp;amp;gt;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#ifdef _WIN32&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
WSADATA wsa; // Winsock data&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* printUsage function who describe the utilisation of this script.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param char* bin : the name of the current binary.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
void printHeader(char* bin){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[?] Usage : %s &amp;amp;amp;lt;port&amp;amp;amp;gt; [&amp;amp;amp;lt;method&amp;amp;amp;gt; &amp;amp;amp;lt;server_cert&amp;amp;amp;gt; &amp;amp;amp;lt;server_private_key&amp;amp;amp;gt;]\n&amp;amp;amp;quot;, bin);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[?] With &amp;amp;amp;lt;method&amp;amp;amp;gt; :\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t1 :\tTLS v1\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t2 :\tSSL v2 (deprecated so disabled)\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t3 :\tSSL v3\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t4 :\tSSL v2 &amp;amp;amp;amp; v3 (default)\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* makeServerSocket function who create a traditionnal server socket, bind it and listen to it.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int port : the port to listen&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @return int socket : the socket number created&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int makeServerSocket(int port){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int sock;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
struct sockaddr_in addr;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#ifdef _WIN32&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
WSAStartup(MAKEWORD(2,0),&amp;amp;amp;amp;wsa);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
sock = socket(PF_INET, SOCK_STREAM, 0);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
memset(&amp;amp;amp;amp;addr, 0, sizeof(addr));&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
addr.sin_family = AF_INET;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
addr.sin_port = htons(port);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
addr.sin_addr.s_addr = INADDR_ANY;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(bind(sock, (struct sockaddr*)&amp;amp;amp;amp;addr, sizeof(addr)) != 0){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
perror(&amp;amp;amp;quot;[-] Can’t bind port on indicated port…&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(listen(sock, 10) != 0){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
perror(&amp;amp;amp;quot;[-] Can’t listening on indicated port…&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Server listening on the %d port…\n&amp;amp;amp;quot;, port);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return sock;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* callbackGeneratingKey called during internal dynamic key generation.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* A callback function may be used to provide feedback about the&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* progress of the key generation. If callback is not NULL, it will&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* be called as follows:&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – While a random prime number is generated, it is called as&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* described in BN_generate_prime(3).&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – When the n-th randomly generated prime is rejected as not&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* suitable for the key, callback(2, n, cb_arg) is called.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – When a random p has been found with p-1 relatively prime to e,&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* it is called as callback(3, 0, cb_arg).&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* The process is then repeated for prime q with callback(3, 1, cb_arg).&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int p : callback random prime flag&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int n : n-th randomly generation&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param void *arg : argument for the callback passed from initial call&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
static void callbackGeneratingKey(int p, int n, void *arg){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
char c=’B’;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if (p == 0) c = ‘.’; // generating key…&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if (p == 1) c = ‘+’; // near the end of generation…&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if (p == 2) c = ‘*’; // rejecting current random generation…&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if (p == 3) c = ‘\n’; // key generated&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
fputc(c, stderr); // print generation state&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* makekCert function who create the server certificat containing public key and&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* the server private key signed (dynamic method).&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param X509 **x509p : potential previous instance of X509 certificat&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param EVP_PKEY **pkeyp : potential previous instance of private key&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int bits : length of the RSA key to generate (precaunized greater than or equal 2048b)&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int serial : long integer representing a serial number&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int days : number of valid days of the certificat&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @see Inpired from /demos/x509/mkcert.c file of OpenSSL library.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
void makekCert(X509 **x509p, EVP_PKEY **pkeyp, int bits, int serial, int days){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509 *x;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
EVP_PKEY *pk;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
RSA *rsa;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_NAME *name = NULL;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;if((pkeyp == NULL) || (*pkeyp == NULL)){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if((pk = EVP_PKEY_new()) == NULL)&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
} else&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
pk= *pkeyp;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if((x509p == NULL) || (*x509p == NULL)){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if ((x = X509_new()) == NULL)&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
} else&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
x= *x509p;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;// create RSA key&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
rsa = RSA_generate_key(bits, RSA_F4, callbackGeneratingKey, NULL);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(!EVP_PKEY_assign_RSA(pk, rsa))&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
rsa = NULL;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;X509_set_version(x, 2); // why not 3 ?&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ASN1_INTEGER_set(X509_get_serialNumber(x), serial);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_gmtime_adj(X509_get_notBefore(x), 0); // define validation begin cert&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_gmtime_adj(X509_get_notAfter(x), (long)60*60*24*days); // define validation end cert&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_set_pubkey(x, pk); // define public key in cert&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
name = X509_get_subject_name(x);&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;// This function creates and adds the entry, working out the&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// correct string type and performing checks on its length.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// Normally we’d check the return value for errors…&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_NAME_add_entry_by_txt(name, &amp;amp;amp;quot;C&amp;amp;amp;quot;, MBSTRING_ASC, (const unsigned char*)&amp;amp;amp;quot;XX&amp;amp;amp;quot;, -1, -1, 0); // useless if more anonymity needed&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_NAME_add_entry_by_txt(name,&amp;amp;amp;quot;CN&amp;amp;amp;quot;, MBSTRING_ASC, (const unsigned char*)&amp;amp;amp;quot;ASRAT&amp;amp;amp;quot;, -1, -1, 0); // useless if more anonymity needed&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;// Its self signed so set the issuer name to be the same as the subject.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_set_issuer_name(x, name);&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;if(!X509_sign(x, pk, EVP_md5())) // secured more with sha1? md5/sha1? sha256?&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;*x509p = x;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*pkeyp = pk;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* initSSLContext function who initialize the SSL/TLS engine with right method/protocol&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int ctxMethod : the number coresponding to the method/protocol to use&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @return SSL_CTX *ctx : a pointer to the SSL context created&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX* initSSLContext(int ctxMethod){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
const SSL_METHOD *method;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX *ctx;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;SSL_library_init(); // initialize the SSL library&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_load_error_strings(); // bring in and register error messages&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
OpenSSL_add_all_algorithms(); // load usable algorithms&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;switch(ctxMethod){ // create new client-method instance&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case 1 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = TLSv1_server_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use TLSv1 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// SSLv2 isn’t sure and is deprecated, so the latest OpenSSL version on Linux delete his implementation.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
/*case 2 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv2_server_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv2 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case 3 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv3_server_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv3 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case 4 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv23_server_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv2&amp;amp;amp;amp;3 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
default :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv23_server_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv2&amp;amp;amp;amp;3 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;ctx = SSL_CTX_new(method); // create new context from selected method&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(ctx == NULL){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ERR_print_errors_fp(stderr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return ctx;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* loadCertificates function who load private key and certificat from files.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* 3 mecanisms available :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – loading certificate and private key from file(s)&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – use embed hardcoded certificate and private key in the PEM format&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* – generate random and dynamic certificate and private key at each server’s launch instance.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param SSL_CTX* ctx : the SSL/TLS context&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param char *certFile : filename of the PEM certificat&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param char *keyFile : filename of the PEM private key&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
void loadCertificates(SSL_CTX* ctx, const char* certFile, const char* keyFile){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// The server private key in PEM format, if internals required&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
/*const char *keyBuffer = &amp;amp;amp;quot;—–BEGIN PRIVATE KEY—–\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;MIIEvQIBADANBgkqhkiG9w0BAQEFAASCBKcwggSjAgEAAoIBAQDP1SC2T/+NW59H\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;CYF0mzkoFcObGUAkoK7mvemFk2P99FLcKbqYKZZDMLVBg+tLU12kuIefYrC4G8F7\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;K8WReTZ+ZBWI1h+gEBhilZ0O4+XXoww2tjVyuHNe5twSxOhRYvoPNSKMLPR70Oij\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;b4nHSyu0a7JHAWvEdpk7HIeWugKYbY8ss58iCmkWGcrop/od6SPW12W+ugAyDGD9\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;F1Otrmb+T3KQPadlPgGdNprvVXHjk+eS1RcwOsT630usogl1JqhoAT4ViQvxDP0J\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;LEffPvG2Iow2WoRtjLGfKqGinhtrLyuht5s3XBzm05kHYNVDc1vkWPvk4PuoIfTp\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;ezrxuMR5AgMBAAECggEADV6wlAnhbr6OKIu8ADxcGPANfVTKg5Cyr7VX6Hfq3tNw\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;4SjuEAvc1sWzY1uRL29VfttAHkjDBZUDhWDzfMBHeSoHGJ5tumZOq0jkqaiPiKe8\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;iWh/V7n18gz3610vdMzhOUk5x7q8n5p43Mq4GlIDpb+n4Fl/DUxz3xGex1t//z4v\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;W7U1j+dKxiZGaNz2dyVVM7eHaynvEE4QL8i4msjhmrFSItqjF/0M/CJ0oEPPb2VL\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;6GLSfqCcjBzt0Sy93gVNhxO+KjMpumB1a9omDxBkTO4HF4xoDojrtkgYXaUx3uKk\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;Gc35xLoOdkn/pNDDzGzQT+xWYOO6IBxJGj/INvnIAQKBgQDwdVsC02z0Pb4JrlcM\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;KRBGyJetxxQguiZ3TYMIGMMP/fQZn3uofmWxNGPbk20VmDXXtZFsCJG7zrFVOUe3\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;eXIPjE2ho80aPAMWeiPAMkivhj0OnPHTg5sof75uH5F9zPerw7kgcwZMFPZk/Za0\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;53gxjakIZo2mlrtaomZLD/U+2QKBgQDdQ/EQlMG5+sjGn6MQrqpzlIT+PYQ4OmFE\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;p8B6AKtwC1oVKkY/1dWVUQ33DqTbXv8i8zN2mplMaFM/6rJNcY4BhKwBm+pW5XuV\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;LHLMGGkubues3bCb2OHax8DOm/i6hDJ14cEORsZSA2Jt6qzxaQ9HrtCZy29S5FIg\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;cFGCLHNuoQKBgAIe5tiViMZ2rPBk6zueORiGuF+9+712JtSyiE9P+Jhxgu+e6nZH\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;9xmi/qZ3HGUuXHs0jL3JLY/ceM/pm2pQ1eKxOBYO3cY3dUeDeEE/sEhsBKnWVIOr\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;C3lF9yX9fUkAv8ZyCXXxzcJqBOpLGkMqL3Mwbqc2UFWBytE30XMkBuOxAoGBAI8l\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;qGzAwIBwpboShy2AwteZq1zMMaEq68i9+oEzs7X+Mh5lRiOAVPiQAsfmGnOuBsP2\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;sUG3DRxolgtQ7F+76lJDIgC8fSQQvR4qLm6qEEoxCANHPT3mV1/yQWOpdoY8hmTL\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;U9nHogBnHiPcYlygSnlmuJ/3BCONgTBpWeIsndVhAoGAOFpnITiCmUFc5AUaxglZ\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;fz4fC+Mt4SF4XGFUtL8feGN4XGXHU6lQVQqu1yaRpYjSTabq6V6LLvVOh1sb+qZw\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;sSB4hC5C+VjjIBScsaN0pytFdL0+FeRaGPVBUs/yBWzfhi6Lm9vE8ebE0fMxr7b5\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;gw4qJCTvXYDZ8ZOIwG4YRRs=\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;—–END PRIVATE KEY—–\n&amp;amp;amp;quot;;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// The server certificat containing public key in PEM format&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
const char *certBuffer = &amp;amp;amp;quot;—–BEGIN CERTIFICATE—–\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;MIIDiTCCAnGgAwIBAgIJAK0drhMsLqg2MA0GCSqGSIb3DQEBBQUAMFsxCzAJBgNV\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;BAYTAlhYMQowCAYDVQQIDAFYMQowCAYDVQQHDAFYMQowCAYDVQQKDAFYMQowCAYD\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;VQQLDAFYMQowCAYDVQQDDAFYMRAwDgYJKoZIhvcNAQkBFgFYMB4XDTEyMDMwMTEz\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;NDcwM1oXDTEyMDMzMTEzNDcwM1owWzELMAkGA1UEBhMCWFgxCjAIBgNVBAgMAVgx\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;CjAIBgNVBAcMAVgxCjAIBgNVBAoMAVgxCjAIBgNVBAsMAVgxCjAIBgNVBAMMAVgx\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;EDAOBgkqhkiG9w0BCQEWAVgwggEiMA0GCSqGSIb3DQEBAQUAA4IBDwAwggEKAoIB\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;AQDP1SC2T/+NW59HCYF0mzkoFcObGUAkoK7mvemFk2P99FLcKbqYKZZDMLVBg+tL\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;U12kuIefYrC4G8F7K8WReTZ+ZBWI1h+gEBhilZ0O4+XXoww2tjVyuHNe5twSxOhR\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;YvoPNSKMLPR70Oijb4nHSyu0a7JHAWvEdpk7HIeWugKYbY8ss58iCmkWGcrop/od\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;6SPW12W+ugAyDGD9F1Otrmb+T3KQPadlPgGdNprvVXHjk+eS1RcwOsT630usogl1\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;JqhoAT4ViQvxDP0JLEffPvG2Iow2WoRtjLGfKqGinhtrLyuht5s3XBzm05kHYNVD\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;c1vkWPvk4PuoIfTpezrxuMR5AgMBAAGjUDBOMB0GA1UdDgQWBBRG76BYshU93k3q\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;hy6gIpMl/VUDhTAfBgNVHSMEGDAWgBRG76BYshU93k3qhy6gIpMl/VUDhTAMBgNV\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;HRMEBTADAQH/MA0GCSqGSIb3DQEBBQUAA4IBAQBCGmmyVt9gRJ0fuWh9o5MnT70m\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;nwbt0fM3Z6AO/Gkc0fkc6H4pZ3tnEtubtXBBm24wMFfXutcXFAjZMk0OTCPj5U8I\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;0/yjk5zuBdgktIFUTjs4Os/Ct2wvIfIiOm/WeL3FZOWli/HOX1PqjbeF/HXN+069\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;31U++ajDzM0uDFGc7dEPTXTEuE7w81696n9PTF0PSLt3/xIOwkMx28Wykc9XKgAp\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;MztGxeEtyb32ib+zL7UhEyuDHnW4haC8QsjG1QLpESTMMASbRe6QxrYxuMFjkf+g\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;FMw9jUYsThZropV2gFipcltT63ncyk0/W8gj1zmF6QsC46r1MFPUfnc/I6dx\n&amp;amp;amp;quot;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
&amp;amp;amp;quot;—–END CERTIFICATE—–\n&amp;amp;amp;quot;;*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509 *cert = NULL;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
EVP_PKEY *pkey = NULL;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// RSA *rsa = NULL; // if internal private key and certificat required&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
//BIO *cbio, *kbio; // if internal private key and certificat required&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;if(certFile == NULL || keyFile == NULL){&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/*&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// if internal certificat and private key required&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[*] Loading internal server’s certificat and private key.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
cbio = BIO_new_mem_buf((void*)certBuffer, -1);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
PEM_read_bio_X509(cbio, &amp;amp;amp;amp;cert, 0, NULL);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX_use_certificate(ctx, cert);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
kbio = BIO_new_mem_buf((void*)keyBuffer, -1);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
PEM_read_bio_RSAPrivateKey(kbio, &amp;amp;amp;amp;rsa, 0, NULL);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX_use_RSAPrivateKey(ctx, rsa);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;printf(&amp;amp;amp;quot;[*] Generate random server’s certificat and private key.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
makekCert(&amp;amp;amp;amp;cert, &amp;amp;amp;amp;pkey, 2048, 0, 0);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX_use_certificate(ctx, cert);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX_use_PrivateKey(ctx, pkey);&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;// set the local certificate from certFile if certFile specified&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// set the private key from keyFile (may be the same as certFile) if specified&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
} else if(SSL_CTX_use_certificate_file(ctx, certFile, SSL_FILETYPE_PEM) &amp;amp;amp;lt;= 0 ||&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX_use_RSAPrivateKey_file(ctx, keyFile, SSL_FILETYPE_PEM) &amp;amp;amp;lt;= 0){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ERR_print_errors_fp(stderr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
} else&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[*] Server’s certificat and private key loaded from file.\n&amp;amp;amp;quot;);&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;// verify private key match the public key into the certificate&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(!SSL_CTX_check_private_key(ctx)){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
fprintf(stderr, &amp;amp;amp;quot;[-] Private key does not match the public certificate…\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
} else&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Server’s private key match public certificat !\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* showCerts function who catch and print out certificate’s data from the client.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param SSL* ssl : the SSL/TLS connection&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
void showCerts(SSL* ssl){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509 *cert;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
char *subject, *issuer;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;cert = SSL_get_peer_certificate(ssl); // get the client’s certificate&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(cert != NULL){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
subject = X509_NAME_oneline(X509_get_subject_name(cert), 0, 0); // get certificate’s subject&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
issuer = X509_NAME_oneline(X509_get_issuer_name(cert), 0, 0); // get certificate’s issuer&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;printf(&amp;amp;amp;quot;[+] Client certificates :\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\tSubject: %s\n&amp;amp;amp;quot;, subject);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\tIssuer: %s\n&amp;amp;amp;quot;, issuer);&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;free(subject); // free the malloc’ed string&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
free(issuer); // free the malloc’ed string&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_free(cert); // free the malloc’ed certificate copy&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
else&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[-] No client’s certificates\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* routine function who treat the content of data received and reply to the client.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* this function is threadable and his context sharedable.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param SSL* ssl : the SSL/TLS connection&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
void routine(SSL* ssl){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
char buf[1024], reply[1024];&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int sock, bytes;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
const char* echo = &amp;amp;amp;quot;Enchante %s, je suis ServerName.\n&amp;amp;amp;quot;;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;if(SSL_accept(ssl) == -1) // accept SSL/TLS connection&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ERR_print_errors_fp(stderr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
else{&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Cipher used : %s\n&amp;amp;amp;quot;, SSL_get_cipher(ssl));&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
showCerts(ssl); // get any client certificates&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
bytes = SSL_read(ssl, buf, sizeof(buf)); // read data from client request&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(bytes &amp;amp;amp;gt; 0){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
buf[bytes] = 0;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Client data received : %s\n&amp;amp;amp;quot;, buf);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
sprintf(reply, echo, buf); // construct response&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_write(ssl, reply, strlen(reply)); // send response&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
} else {&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
switch(SSL_get_error(ssl, bytes)){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case SSL_ERROR_ZERO_RETURN :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;SSL_ERROR_ZERO_RETURN : &amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case SSL_ERROR_NONE :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;SSL_ERROR_NONE : &amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case SSL_ERROR_SSL:&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;SSL_ERROR_SSL : &amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ERR_print_errors_fp(stderr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
sock = SSL_get_fd(ssl); // get traditionnal socket connection from SSL connection&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_shutdown(ssl);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_free(ssl); // release SSL connection state&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
CLOSESOCKET(sock); // close socket&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* main function who coordinate the socket and SSL connection creation, then receive and emit data to and from the client.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int main(int argc, char **argv){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int sock, ctxMethod, port;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX *ctx;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
const char *certFile, *keyFile;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;if(argc != 2 &amp;amp;amp;amp;&amp;amp;amp;amp; argc != 5){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printHeader(argv[0]);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
exit(0);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;port = (atoi(argv[1]) &amp;amp;amp;gt; 0 &amp;amp;amp;amp;&amp;amp;amp;amp; atoi(argv[1]) &amp;amp;amp;lt; 65535) ? atoi(argv[1]) : DEFAULT_PORT;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ctxMethod = (argc &amp;amp;amp;gt;= 3) ? atoi(argv[2]) : 4; // SSLv2, SSLv3, SSLv2&amp;amp;amp;amp;3 or TLSv1&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ctx = initSSLContext(ctxMethod); // load SSL library and dependances&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
certFile = (argc &amp;amp;amp;gt;= 4) ? argv[3] : NULL;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
keyFile = (argc &amp;amp;amp;gt;= 5) ? argv[4] : NULL;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;loadCertificates(ctx, certFile, keyFile); // load certificats and keys&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;sock = makeServerSocket(port); // make a classic server socket&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;while(42){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
struct sockaddr_in addr;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL *ssl;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SOCKLEN_T len = sizeof(addr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int client = accept(sock, (struct sockaddr*)&amp;amp;amp;amp;addr, &amp;amp;amp;amp;len); // accept connection of client&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Connection [%s:%d]\n&amp;amp;amp;quot;, inet_ntoa(addr.sin_addr), ntohs(addr.sin_port));&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ssl = SSL_new(ctx); // get new SSL state with context&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_set_fd(ssl, client); // set traditionnal socket to SSL&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
routine(ssl); // apply routine to the socket’s content&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;CLOSESOCKET(sock); // close socket&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#ifdef _WIN32&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
WSACleanup(); // Windows’s Winsock clean&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX_free(ctx); // release SSL’s context&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return 0;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;[/c]
Fonctionnalités du client :
- Se connecte à un serveur sur un port défini
- Utilise la version du protocole au choix entre SSL2.0, SSL3.0n SSL2.0 & 3.0, TLS1.0
- Envoi un message au serveur et attend le retour de celui-ci.
* SSL/TLS client demonstration. This source code is cross-plateforme Windows and Linux.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* Compile under Linux with : g++ main.cpp -Wall -lssl -lcrypto -o main&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* Usage :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* # run the client to 127.0.0.1 on port 1337 for SSLv2&amp;amp;amp;amp;3 protocol&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* $ [./]client[.exe] 127.0.0.1 1337&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* # run the client to 127.0.0.1 on port 1337 for TLSv1 protocol&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* $ [./]client[.exe] 127.0.0.1 1337 1&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @author x@s&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#define DEFAULT_PORT 443&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#ifdef __unix__ // __unix__ is usually defined by compilers targeting Unix systems&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;unistd.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;sys/socket.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;resolv.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;netdb.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define SOCKLEN_T socklen_t&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define CLOSESOCKET close&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#elif defined _WIN32 // _Win32 is usually defined by compilers targeting 32 or 64 bit Windows systems&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;windows.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# include &amp;amp;amp;lt;winsock2.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define SOCKLEN_T int&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# define CLOSESOCKET closesocket&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#include &amp;amp;amp;lt;stdio.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;errno.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;malloc.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;string.h&amp;amp;amp;gt;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#include &amp;amp;amp;lt;openssl/crypto.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/x509.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/pem.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/ssl.h&amp;amp;amp;gt;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#include &amp;amp;amp;lt;openssl/err.h&amp;amp;amp;gt;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;#ifdef _WIN32&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
WSADATA wsa; // Winsock data&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* printUsage function who describe the utilisation of this script.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param char* bin : the name of the current binary.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
void printHeader(char* bin){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[?] Usage : %s &amp;amp;amp;lt;hostname&amp;amp;amp;gt; &amp;amp;amp;lt;port&amp;amp;amp;gt; [&amp;amp;amp;lt;method&amp;amp;amp;gt;]\n&amp;amp;amp;quot;, bin);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[?] With optional &amp;amp;amp;lt;method&amp;amp;amp;gt; :\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t1 :\tTLS v1\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t2 :\tSSL v2 (deprecated so disabled)\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t3 :\tSSL v3\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\t4 :\tSSL v2 &amp;amp;amp;amp; v3 (default)\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* makeClientSocket function who create a traditionnal client socket to the hostname throught the port.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param char* hostname : the target to connect to&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int port : the port to connect throught&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @return int socket ; the socket number created&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int makeClientSocket(const char *hostname, int port){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int sock;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
struct hostent *host;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
struct sockaddr_in addr;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#ifdef _WIN32&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
WSAStartup(MAKEWORD(2,0),&amp;amp;amp;amp;wsa);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if((host = gethostbyname(hostname)) == NULL ){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
perror(hostname);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
sock = socket(PF_INET, SOCK_STREAM, 0);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
memset(&amp;amp;amp;amp;addr, 0, sizeof(addr));&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
addr.sin_family = AF_INET;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
addr.sin_port = htons(port);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
addr.sin_addr.s_addr = *(long*)(host-&amp;amp;amp;gt;h_addr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(connect(sock, (struct sockaddr*)&amp;amp;amp;amp;addr, sizeof(addr)) != 0){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
CLOSESOCKET(sock);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
perror(hostname);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return sock;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* initSSLContext function who initialize the SSL/TLS engine with right method/protocol&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param int ctxMethod : the number coresponding to the method/protocol to use&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @return SSL_CTX *ctx ; a pointer to the SSL context created&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX* initSSLContext(int ctxMethod){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
const SSL_METHOD *method;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX *ctx;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;SSL_library_init(); // initialize the SSL library&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_load_error_strings(); // bring in and register error messages&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
OpenSSL_add_all_algorithms(); // load usable algorithms&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;switch(ctxMethod){ // create new client-method instance&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case 1 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = TLSv1_client_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use TLSv1 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// SSLv2 isn’t sure and is deprecated, so the latest OpenSSL version delete his implementation.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
/*case 2 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv2_client_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv2 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case 3 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv3_client_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv3 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
case 4 :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv23_client_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv2&amp;amp;amp;amp;3 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
break;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
default :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
method = SSLv23_client_method();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Use SSLv2&amp;amp;amp;amp;3 method.\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;ctx = SSL_CTX_new(method); // create new context from selected method&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(ctx == NULL){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ERR_print_errors_fp(stderr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
abort();&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return ctx;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* showCerts function who catch and print out certificat’s data from the server&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* @param SSL* ssl : the SSL/TLS connection&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
void showCerts(SSL* ssl){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509 *cert;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
char *subject, *issuer;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;cert = SSL_get_peer_certificate(ssl); // get the server’s certificate&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(cert != NULL){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
subject = X509_NAME_oneline(X509_get_subject_name(cert), 0, 0); // get certificat’s subject&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
issuer = X509_NAME_oneline(X509_get_issuer_name(cert), 0, 0); // get certificat’s issuer&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;printf(&amp;amp;amp;quot;[+] Server certificates :\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\tSubject: %s\n&amp;amp;amp;quot;, subject);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;\tIssuer: %s\n&amp;amp;amp;quot;, issuer);&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;free(subject); // free the malloc’ed string&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
free(issuer); // free the malloc’ed string&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
X509_free(cert); // free the malloc’ed certificate copy&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
if(SSL_get_verify_result(ssl) == X509_V_OK) // check certificat’s trust&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Server certificates X509 is trust!\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
else&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[-] Server certificates X509 is not trust…\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
else&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[-] No server’s certificates\n&amp;amp;amp;quot;);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;/**&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
* main function who coordinate the socket and SSL connection creation, then receive and emit data to and from the server.&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
*/&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int main(int argc, char **argv){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
int sock, bytes, ctxMethod, port;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX *ctx;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL *ssl;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
char buf[1024];&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
char *hostname;&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;if(argc != 3 &amp;amp;amp;amp;&amp;amp;amp;amp; argc != 4){&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printHeader(argv[0]);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
exit(0);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;hostname = argv[1];&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
port = (atoi(argv[2]) &amp;amp;amp;gt; 0 &amp;amp;amp;amp;&amp;amp;amp;amp; atoi(argv[2]) &amp;amp;amp;lt; 65535) ? atoi(argv[2]) : DEFAULT_PORT;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ctxMethod = (argc == 4) ? atoi(argv[3]) : 4; // SSLv2, SSLv3, SSLv2&amp;amp;amp;amp;3 or TLSv1&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ctx = initSSLContext(ctxMethod); // load SSL library and dependances&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
sock = makeClientSocket(hostname, port); // make a classic socket to the hostname throught the port&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ssl = SSL_new(ctx); // create new SSL connection state&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;SSL_set_fd(ssl, sock); // attach the socket descriptor&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;if(SSL_connect(ssl) == -1) // make the SSL connection&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
ERR_print_errors_fp(stderr);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
else{&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_set_mode(ssl, SSL_MODE_AUTO_RETRY); // if the server suddenly wants a new handshake,&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// OpenSSL handles it in the background. Without this&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// option, any read or write operation will return an&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
// error if the server wants a new handshake.&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;char msg[] = &amp;amp;amp;quot;ClientName&amp;amp;amp;quot;;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Cipher used : %s\n&amp;amp;amp;quot;, SSL_get_cipher(ssl));&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
showCerts(ssl); // get any certificats&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_write(ssl, msg, strlen(msg)); // encrypt and send message&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
bytes = SSL_read(ssl, buf, sizeof(buf)); // get response and decrypt content&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
buf[bytes] = 0;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
printf(&amp;amp;amp;quot;[+] Server data received : %s\n&amp;amp;amp;quot;, buf);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_shutdown(ssl);&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_free(ssl); // release SSL connection state&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;}&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
CLOSESOCKET(sock); // close socket&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#ifdef _WIN32&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
WSACleanup(); // Windows’s Winsock clean&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
#endif&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
SSL_CTX_free(ctx); // release SSL’s context&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
return 0;&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
}&amp;lt;/p&amp;gt;&lt;br /&gt;<br />
&amp;lt;p&amp;gt;[/c]
L’objectif de ce client/serveur est de disposer d’un code source d’exemple fonctionnel, cross-plateformes, épuré, documenté et clair quand à la mise en place d’une connexion SSL/TLS en C pour un quelconque développement futur.
La création du certificat et de la clé privée du serveur peut se faire avec la ligne de commande suivante (résultat dans un unique fichier) :
[bash]openssl req -x509 -nodes -newkey rsa:2048 -keyout server.pem -out server.pem[/bash]
Compilation sous environnements Linux:
[bash]g++ main.cpp -Wall -lssl -lcrypto -o main[/bash]
Sous Windows, OpenSSL doit être installé avec les fichiers d’en-têtes disponibles dans les includes de l’IDE. Le linkerde l’IDE utilisé doit utiliser les bibliothèques statiques d’OpenSSL (libcrypto.a et libssl.a) ou bien les DLL d’OpenSSL (libeay32.dll et libssl32.dll) doivent être présentes au côté du binaire.
Utilisation du serveur:
[bash]&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# Usage :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# run the server on port 1337 for SSLv2&amp;amp;amp;amp;3 protocol with internal randomly generated key and certificate&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
$ [./]server[.exe] 1337&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# run the server on port 1337 for TLSv1 protocol with key and certificate in server.pem file&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
$ [./]server[.exe] 1337 1 server.pem server.pem&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
[/bash]
Utilisation du client:
[bash]&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# Usage :&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# run the client to 127.0.0.1 on port 1337 for SSLv2&amp;amp;amp;amp;3 protocol&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
$ [./]client[.exe] 127.0.0.1 1337&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
# run the client to 127.0.0.1 on port 1337 for TLSv1 protocol&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
$ [./]client[.exe] 127.0.0.1 1337 1&amp;lt;br /&amp;gt;&lt;br /&gt;<br />
[/bash]
L’ensemble des sources et binaires (Gcc et Code::Blocks) sont disponibles dans l’archive suivante [Client/Server OpenSSL]. La version utilisée d’OpenSSL au temps de développement était la 1.0.0e.